Multiple comparison correction (MCC)#
In this notebook, we’ll continue with the topic of multiple comparison correction (MCC).
What you’ll learn: after this lab, you’ll …
know the relative advantages and disadvantages of different MCC techniques
Estimated time needed to complete: 1 hour
Credits: This notebook is based on a blog by Matthew Brett and a previous Matlab-based lab by H. Steven Scholte.
Why do we need MCC?#
Univariate analyses of fMRI data essentially test hypotheses about your data (operationalized as contrasts between your \(\hat{\beta}\) estimates) for each voxel separately. So, in practice, given that the MNI (2 mm) standard template brain contains about 260,000 voxels, you’re conducting 260,000 different statistical tests! The obvious problem, here, is that some tests might turn out significant, while they in fact do not contain any (task-related) activity: the result is driven just by chance.
As a researcher, you should strive to “filter out” the results which are driven by noise (false positives) and keep the results which are actually driven by the true effect (true positives) as much as possible. It turns out that the more tests you do, the larger the chance is that you will find one or more false positives. To deal with this, researchers often employ techniques for multiple comparison correction (MCC): correcting for the inflated chance of false positives when you have multiple tests (comparisons).
In this tutorial, we will walk you through an example (simulated) dataset on which different MCC techniques are employed. We’ll focus on how these different techniques influence the chance for finding false positives.
The example#
We’ll work with the (simulated) group-level results of a hypothetical fMRI experiment. Suppose that the subjects in our hypothetical experiment were shown pictures of cats in the scanner, because we (the experimenters) were interested in which voxels would (de)activate significantly in reponse to these cat pictures (i.e. a contrast of the cat-picture-condition against baseline).
An example of an image shown to the subjects:
After extensive preprocessing, we fitted first-level models in which we evaluated the cat-against-baseline contrast, in which the \(t\)-statistic refers to how strongly each voxel responded to the pictures of cats. After doing a proper group-level analysis, we now have a group-level \(t\)-statistic map, reflecting whether voxels on average (de)activated in response to the pictures of cats.
YOUR ANSWER HERE
The data#
Usually, your whole-brain group-level results are 3D \(z\)- or \(t\)-statictic maps of the size of a standard brain (usually the MNI 2mm template, which has about 260,000 voxels). Plotting in 3D, however, is incredibly cumbersome, so for the sake of the example, we’ll assume that our group-level results are represented as a 2D \(z\)-statistic map, with dimensions \(200 \times 200\). So, we’ll pretend we analyzed the results based on a 2D brain with \(200 \times 200\) “voxels”.
Because we work with simulated data, we can actually specify the “true effect”. In reality, we never know this of course! We are going to assume that there is a small “blob” of voxels in the middle of our “brain” that activates reliably to pictures of cats (with a \(z\)-value of 5.5). This blob is therefore the true effect in our simulation.
Let’s first simulate the data.
import numpy as np
# You don't have to understand how this simulation works exactly
k = 200 # number of vox in each dimension
signal = np.zeros((k, k))
r = 10 # middle of the image
a, b = k // 2, k // 2 # width and height of the circle
y, x = np.ogrid[-a:k-a, -b:k-b]
mask = x * x + y * y <= r * r
signal[mask] = 5.5 # amplitude of effect!
print("Shape of statistic map: %s" % (signal.shape,))
Shape of statistic map: (200, 200)
Alright, now let’s plot the true effect as a 2D image. We’ll define a custom function for this, plot_sim_brain
, to save us some work later.
import matplotlib.pyplot as plt
def plot_sim_brain(brain, mask=None, vmin=-7, vmax=7, cmap='seismic', title='', label='Z-value'):
""" Plots an image of a simulated 2D 'brain' with statistic values, which may be 'masked'.
Parameters
----------
brain : numpy array
A 2D numpy array with statistics
mask : numpy array (or None)
A 2D numpy array with booleans (True = do plot, False = do not plot). If None,
the 'brain' is not masked.
vmin : int/float
Minimum value of colorbar
vmax : int/float
Maximum value of colorbar
cmap : str
Name of colormap to use
title : str
Title of plot
label : str
Label for colorbar
"""
brainm = brain.copy()
if mask is not None: # threshold!
brainm[~mask] = 0
plt.figure(figsize=(8, 10))
plt.imshow(brainm, vmin=vmin, vmax=vmax, aspect='auto', cmap=cmap)
plt.axis('off')
plt.title(title, fontsize=25)
cb = plt.colorbar(orientation='horizontal', pad=0.05)
cb.set_label(label, fontsize=20)
plt.show()
plot_sim_brain(signal, title="True effect")
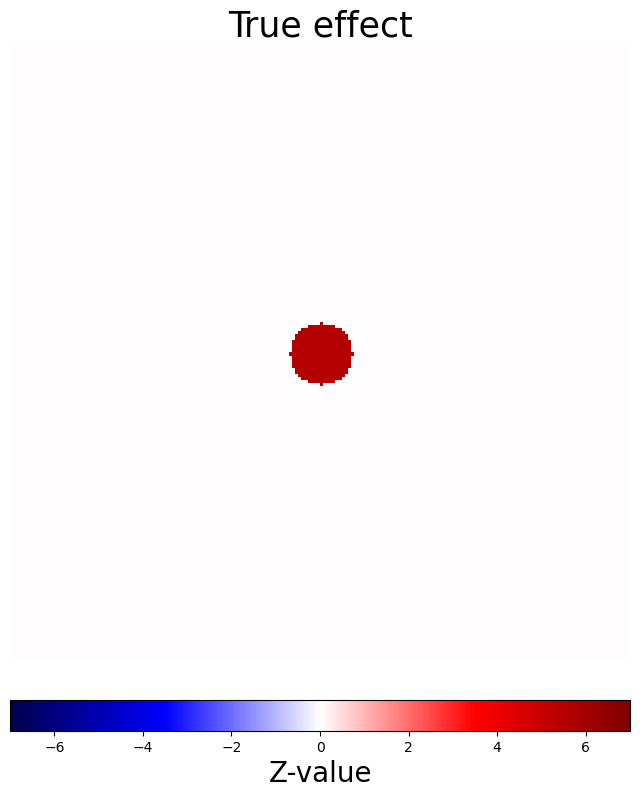
Now, due to the inherent spatial smoothness of fMRI, this particular manifestation of the effect is not very realistic. In particular, the sharp “edges” of the effect are unlikely to occur in real fMRI data. Therefore, to make it a little more realistic, we can spatially smooth the “true effect map”! We will use the gaussian_filter
function (from scipy.ndimage
) with a FWHM of 12 “voxels”.
from scipy.ndimage import gaussian_filter
fwhm = 12
# Convert FWHM to sigma
sigma = fwhm / np.sqrt(8 * np.log(2))
signal_smooth = gaussian_filter(signal, sigma=sigma)
plot_sim_brain(signal_smooth, title="True effect (smooth)")
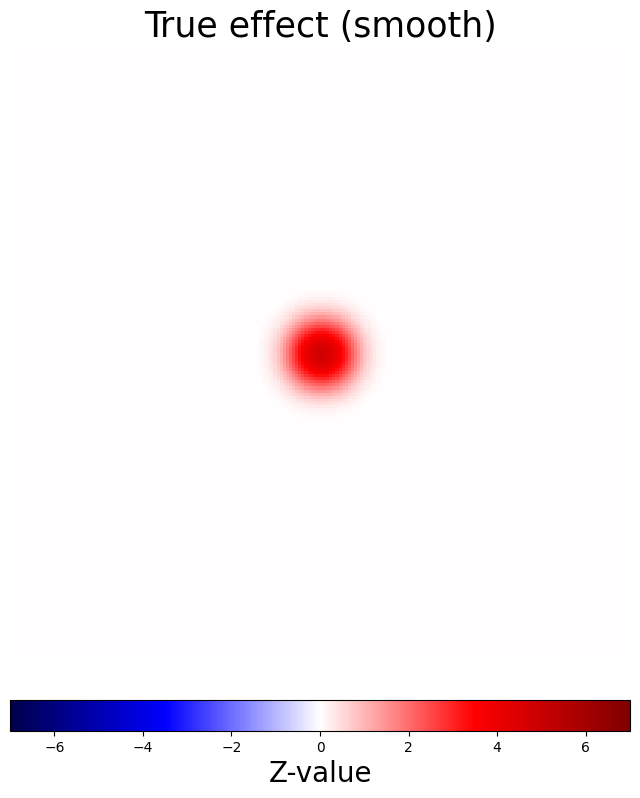
As you’ve learned in the past weeks, the chances are very slim that you’ll find such a “crisp” (true) effect as shown above; often, you might observe significant voxels that are not driven by a true effect, but by (spurious) noise, reflecting false positives.
So, let’s make our data a little more realistic by simulating some random noise, sampled from a normal distribution with mean 0 and a standard deviation of 1. Importantly, we are also going to smooth our noise with the same gaussian filter (with FWHM = 12):
np.random.seed(2) # for reproducibility
noise = np.random.normal(0, 1, size=signal.shape)
noise = gaussian_filter(noise, sigma=sigma)
noise = noise / noise.std()
plot_sim_brain(noise, title='The noise')
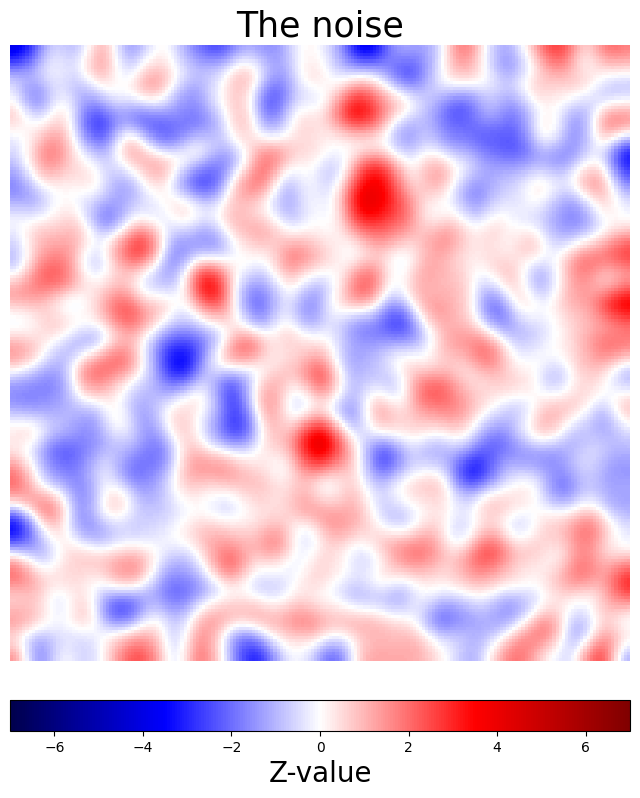
Now, to complete our simulation, we’ll simply add the signal and the noise together (we’ll call this variable data
).
data = signal_smooth + noise
plot_sim_brain(data, title='The data!')
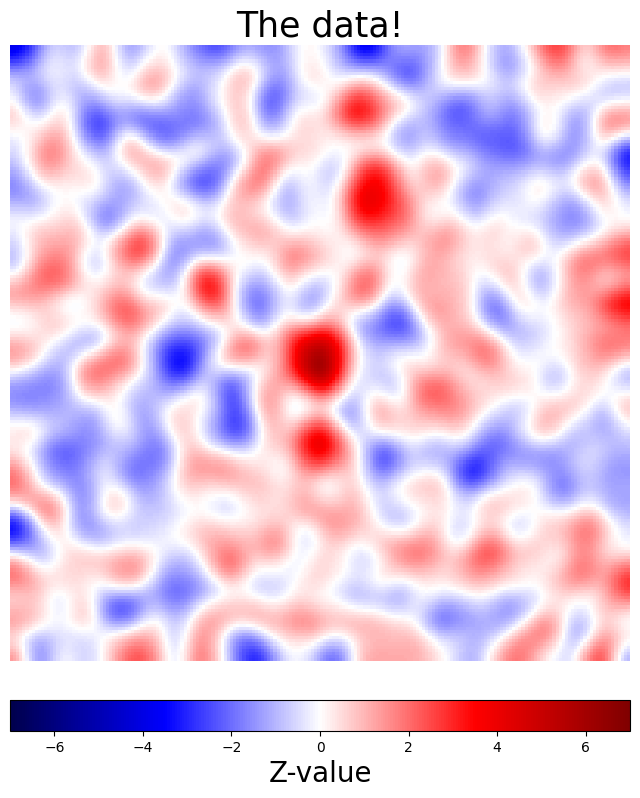
The plot above now represents our simulated data, which contains both a true signal (the “blob” in the middle) and some (spatially correlated) noise. As a researcher, you aim to threshold your data in such a way that you maximize the chance of finding your true signal (true positive effects) and minimize the chance of erroneously treating noise as significant effects (false positive effects).
Uncorrected statistics maps#
In the early days of fMRI analyses, the extent of the MCC problem (more tests = more false positives) was not yet widely known. What researchers simply did was to calculate the \(p\)-values corresponding to the \(z\)-value (or \(t\)-value) maps and threshold those \(p\)-values using some fixed cutoff (“alpha value”), usually 0.05 or 0.01.
To implement this, we can convert all our \(z\)-values to \(p\)-values, compute a “mask” (i.e., an array with True
and False
values, indicating which “voxels” survive the threshold and which do not), and set all “voxels” outside the mask to 0.
Let’s choose a significance level (\(\alpha\)) of 0.05.
alpha = 0.05
Now, let’s convert the \(z\)-values (in the variable data
) to \(p\)-values. We’ll use the stats.norm.sf
function from the scipy
package for this. (This is the same type of function — a “survival function” — that we used to calculate the \(p\)-values corresponding to \(t\)-values before, but this time we use it for \(z\)-values)
# This line converts the z-values to p-values
from scipy import stats
data_pvals = stats.norm.sf(data)
# Implement your ToDo here
# YOUR CODE HERE
raise NotImplementedError()
''' Tests the above ToDo. '''
from niedu.tests.nii.week_6 import test_nsig_uncorrected
test_nsig_uncorrected(data_pvals, alpha, nsig_uncorrected)
We can create a “mask” by comparing our \(p\)-values to our significance level and we can give this mask to our plotting-function (plot_sim_brain
), which will set all “voxels” outside the mask (i.e., those which are False
in the mask) to 0.
smaller_than_alpha = data_pvals < alpha
# Note that 'smaller_than_alpha' is a 2D numpy array with booleans
plot_sim_brain(data, mask=smaller_than_alpha, title=r'Uncorrected ($p < %.4f$)' % alpha)
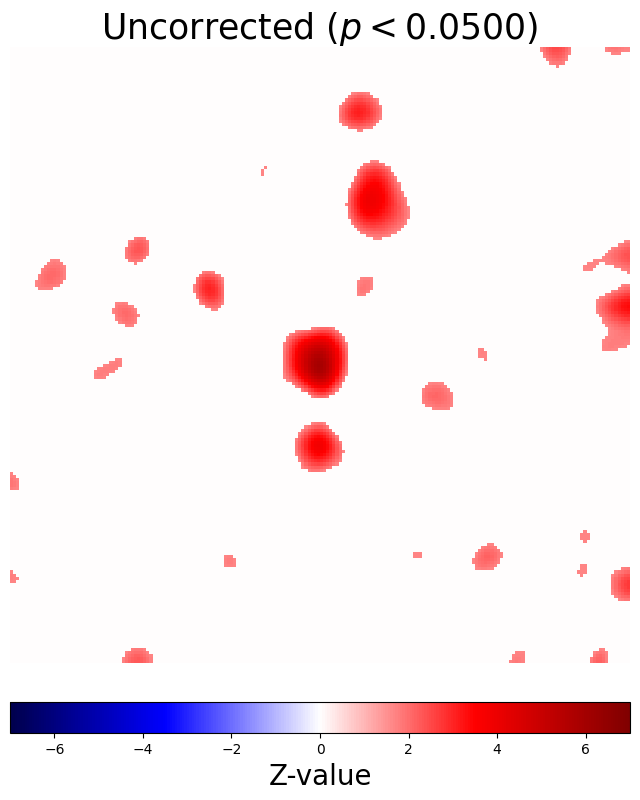
YOUR ANSWER HERE
Bonferroni-correction#
Obviously, given that we know our “true effect”, we can see that the uncorrected results contain a lot of false positives, something that we’d like to avoid! The most obvious way to counter the MCC problem is to adjust the significance level (\(\alpha\)) by the amount of tests we’re performing. Bonferroni correction is such an approach. The way the Bonferroni method does this is by simply dividing the significance level by the amount of tests.
""" Implement the ToDo here. """
# YOUR CODE HERE
raise NotImplementedError()
''' Tests the above ToDo. '''
from niedu.tests.nii.week_6 import test_bonferroni_alpha
test_bonferroni_alpha(alpha, data, bonf_alpha)
This conservative nature of Bonferroni correction, for fMRI at least, is due to the violation of a crucial assumption of Bonferroni correctoin. Which assumption is this, and why does fMRI data/results likely violate this assumption?
YOUR ANSWER HERE
FDR correction#
As you’ve seen so far, uncorrected results tend to be too liberal (too many false positives) and Bonferroni-corrected results are too strict (too many false negatives). The “False Discovery Rate-correction” (FDR) technique is a method to adjust \(p\)-values in a less stringent way. Essentially, while traditional MCC methods (such as Bonferroni) try to control the chance of finding at least one false positive result amongst all your tests (i.e. controlling the “familywise error rate” method), the FDR-method tries to limit the proportion of false positives amongst all your tests which turned out significant. So, if you set your “FDR-proportion” (confusingly also referred to as “alpha”) to 0.05, then it will adjust your initial \(p\)-values such that out of all your significant results, on average 5% will be false positives.
In general, FDR-correction is more sensitive than the Bonferroni correction method (i.e. FDR has a lower type 2 error rate/it is less strict), but if you use it, you do have to accept that about 5% of your (significant) results are false positives!
Now, let’s check out what our results look like after FDR correction:
# fdr implementation from statsmodels package
from fdr import fdrcorrection
alpha_fdr = 0.05 # we use an alpha of 0.05 (5%)
# The fdrcorrection function already returns a "mask"
# Note that it doesn't accept 2D arrays, so we ravel() and then reshape() it
fdr_mask = fdrcorrection(data_pvals.ravel(), alpha=alpha_fdr)[0]
fdr_mask = fdr_mask.reshape(data.shape)
plot_sim_brain(data, mask=fdr_mask, title='FDR correction')
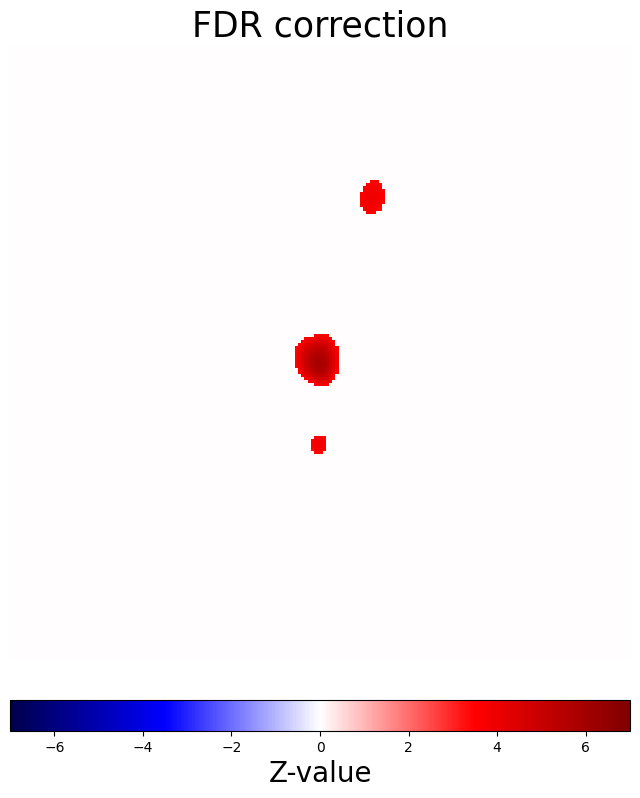
As you can see, the FDR-correction is way more sensitive than the Bonferroni correction (it “recovers” more of the true signal), but it still results in many false positives (but not as many as uncorrected data).
RFT-based correction#
As you’ve seen in the previous examples, it’s quite hard to pick a significance level that strikes a good balance between type 1 errors and type 2 errors, or, phrased differently, between sensitivity (with respect to discovering the true signal) and specificy (i.e. how many of our significant voxels are driven by a true effect).
Let’s go back to the results of the Bonferroni correction. We’ve seen that the results are extremely conservative (few false positives, but many false negatives, i.e. large type 2 error). The major reason for this is that the correction assumes that each test is independent, but in our simulation (and in any fMRI dataset), we know that there exists spatial correlation, meaning that our tests are not independent. In other words, if we know that a certain voxel is signficant in a certain test, it is quite likely that the voxel directly next (or above/below) to it is also significant. Therefore, spatially correlated fMRI statistic maps violate Bonferroni’s assumption of independent tests (this is also the answer to the ToThink from earlier).
As a possible solution to this problem, neuroscientists have developed a method — random field theory — that allows for multiple comparison correction (using FWER) that “corrects” for the smoothness in our data and thresholds accordingly.
Importantly, RFT-correction can either be performed at the voxel-level (testing whether the amplitude, i.e., height of the statistic of a voxel is significant, given the smoothness of the data) and at the cluster-level (testing whether the size of a cluster of voxels is significantly large, given the smoothness of the data). We’ll start with voxel-level RFT.
Voxel-level RFT#
Voxel-level RFT allows for “smoothness-adjusted” thresholding for individual voxels. It does so by assuming a particular distribution for the number of clusters (or “blobs”) one would observe given (1) a particular initial threshold and (2) the smoothness of the data, assuming there is no effect (i.e., the null hypothesis is true). This expected “number of blobs” after thresholding is known as the Euler characteristic. And for standard normal data (i.e., \(z\)-statistics), the expected Euler characteristic is computed as:
where \(R\) refers to the number of “resels” (a number that depends on the smoothness of your data, which we’ll discuss in a bit) and \(z\) refers to the \(z\)-value that you use as an initial threshold. In code, this is:
def expected_EC(z, n_resel):
""" Computes the expected Euler Characteristic for a given number of resels
and initial z-value cutoff.
Parameters
----------
z : int/float or array of int/float
Initial z-value cutoff (can be array)
n_resel : int/float
Number of "resels"
"""
return n_resel * (4 * np.log(2)) * (2 * np.pi) ** (-(2/3)) * z * np.exp(-0.5 * z ** 2)
Importantly, suppose for now that the number of resels is 1000. Then, we can get the expected number of “blobs” in our data for a given \(z\)-value threshold, let’s say \(z = 3\), as follows:
zthresh = 3
n_blobs = expected_EC(z=zthresh, n_resel=1000)
print("For a z-threshold of %i, we expect %.2f blobs in random 2D data with 100 resels." % (zthresh, n_blobs))
For a z-threshold of 3, we expect 27.14 blobs in random 2D data with 100 resels.
We can also evaluate the expected EC for a range of potential \(z\)-value thresholds (e.g., from 0-5) and plot it:
zx = np.linspace(0, 5, 100) # 100 values between 0 and 5
ecs = expected_EC(zx, n_resel=1000) # expected EC also works for multiple z-values at once
plt.figure(figsize=(10, 4))
plt.plot(zx, ecs)
plt.ylabel('Expected EC', fontsize=20)
plt.xlabel('Z-value threshold', fontsize=20)
plt.grid()
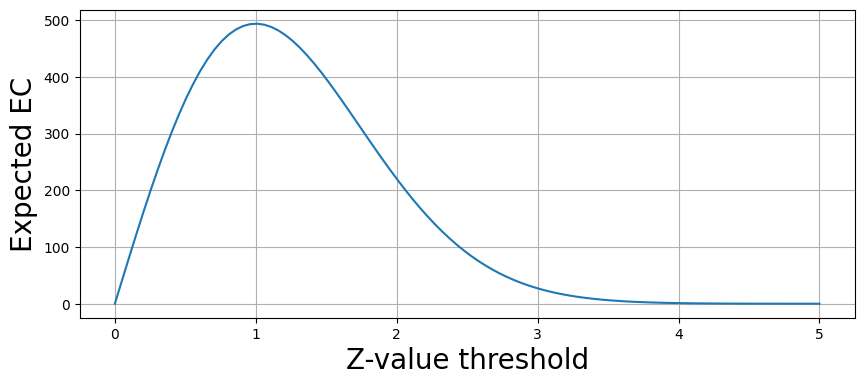
To compute the Euler characteristic, we first need to know how to estimate the number of “resels” for our data. You can think of the number of resels as the number of truly independent elements in your data (“resel” is short for “RESolution ELement”). The number of resels is usually estimated by dividing the number of voxels by the estimated size of the resel. For our simulated 2D data, the number of resels is defined as follows:
where \(N_{X}\) is the number of “voxels” in the first dimension and \(N_{Y}\) the number of “voxels” in the second dimension, and where the resel size (\(\mathrm{size}_{resel}\)) is estimated as the product of the smoothness of our data in all dimensions, measured in FWHM:
So, given a particular size of our resel, \(N_{resel}\) represents how many resels there would “fit” in our data.
# Implement your ToDo here
# YOUR CODE HERE
raise NotImplementedError()
''' Tests the above ToDo. '''
from niedu.tests.nii.week_6 import test_n_resel
test_n_resel(data, n_resel)
Now, another way to interpret EC values is as \(p\)-values: the chance of finding one or more “blobs” for a given \(z\)-value! This way, we can choose a particular \(z\)-value threshold that would correspond to \(p = 0.05\). We do this below:
ecs = expected_EC(zx, 278)
# find the index of the EC value closest to alpha
idx_z = np.abs(ecs - alpha).argmin()
# Index the z-values with idx_z
z_thresh = zx[idx_z]
print("The z-value threshold corresponding to p = 0.05: %.3f" % z_thresh)
The z-value threshold corresponding to p = 0.05: 4.444
YOUR ANSWER HERE
Cluster-level RFT#
In all the previous MCC techniques, we have used voxel-level corrections, which resulting \(p\)-values tell us something about whether the height of a voxel’s statistic (often referred to as “amplitude”) is higher than would be expected under the null-hypothesis. Basically, because we investigated per voxel whether its value is higher than expected, we are making inferences on the level of voxels.
Another type of inference is cluster-level inference, in which you do not test the voxel amplitude, but the size of clusters. Basically, in this type of cluster-extent testing, you are investigating whether the size of the clusters you find are (significantly) larger than to be expected under the null-hypothesis (i.e., no effect).
However, as you can imagine, the null-distribution of cluster sizes (i.e. the size of “significant” clusters you’d expect by chance alone) depends strongly on the initial smoothness of your data. Again: RFT to the rescue!
Basically, RFT can also give us the \(p\)-value for clusters, given their size, by estimating the null-distribution of cluster-sizes based on the data’s smoothness. So, instead of giving us the \(p\)-value for voxels based on the height of their value and the data’s smoothness (i.e., voxel-level RFT), RFT can also do this on the cluster-level by investigating the \(p\)-value of the size of clusters. See how these two RFT-methods relate to each other? They’re doing the same thing — estimating a null-distribution given the smoothness of the data — but for different things: either for the height of the (\(z\)-)statistic value per voxel (voxel-level RFT) or for the size per cluster (cluster-level RFT).
How RFT does this is way beyond the scope of this course, but we’ll walk you through it conceptually, so that you understand the implications of this technique.
Anyway, a first step in cluster-level RFT is to determine a minimum (cutoff) value for your statistics map, which you can use to evaluate whether there are actually clusters in your data. Let’s look at an example, in which we use a minimum value of 3.0:
min_z = 3
thresh_data = (data > min_z)
plot_sim_brain(data, mask=thresh_data, title=r"Clusters after thresholding at $z$ > 3")
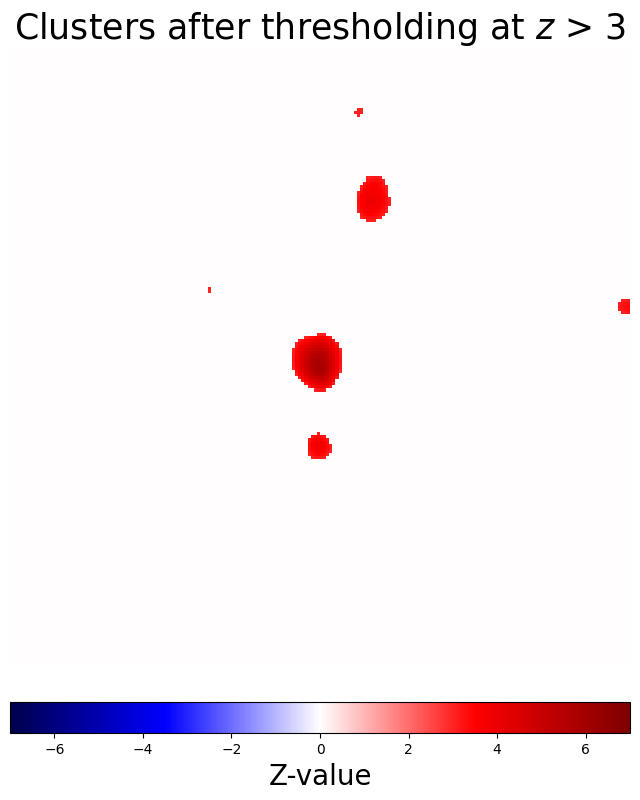
Now, we can use cluster-based RFT to calculate the \(p\)-value for each cluster in the above thresholded data plot. This \(p\)-value reflects the probably of this cluster-size (or larger) under the null-hypothesis. We can then threshold this map with clusters, using a ‘cluster-wise’ \(p\)-value cutoff of 0.01 for example, and plot it again to see how this method affects type 1 and type 2 errors. The function below (threshold_RFT_cluster
) takes three arguments: the statistics-map (our data
variable), a minimum \(z\)-value, and a \(p\)-value cutoff which is used to threshold the clusters.
Below, we do this for a \(z\)-threshold of 3.1 (corresponding to a \(p\)-value of approx. 0.001) and a cluster \(p\)-value threshold of 0.05.
from niedu.utils.nii import rft_cluster_threshold
rft_cl_mask = rft_cluster_threshold(data, z_thresh=3.1, p_clust=0.01)
plot_sim_brain(data, mask=rft_cl_mask, title='RFT thresholding (cluster-based)')
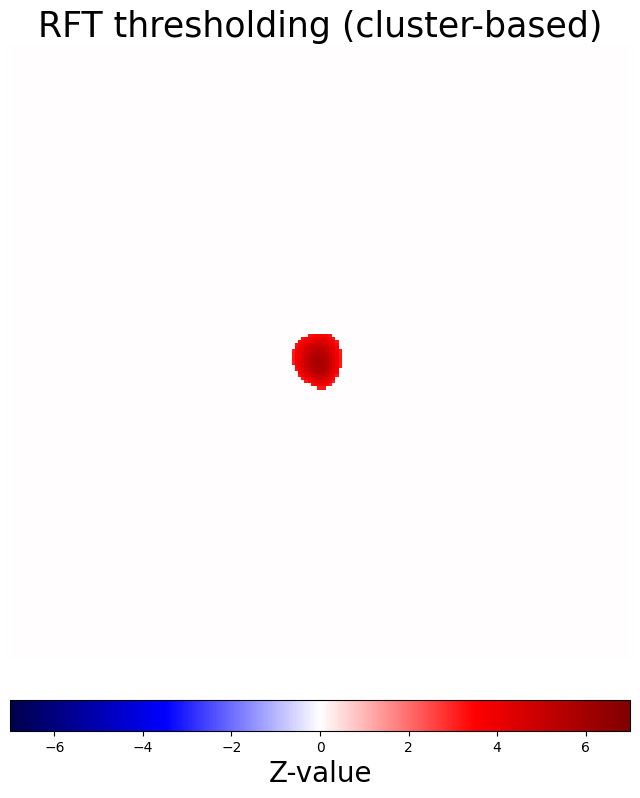
From the above plots, you should see that cluster-thresholding can be a very sensitive way to threshold your data if you expect your effects to occur in relatively large clusters (and given that you’re able to estimate the smoothness of the data appropriately, something that is a topic of debate). As such, it is by far the most used MCC method in univariate fMRI research today (but this does not necessarily mean it’s the best way).
Non-parametric MCC#
In addition to the previously discussed MCC approaches (which are common in parametric group-level models), non-parametric analyses offer another approach. In this approach, the algorithm keeps track of the maximum statistic across permutations. This statistic can refer to the highest voxel-wise amplitude (for voxel-based), largest cluster size (for cluster-based, given some initial \(z\)-value cutoff), or even highest TFCE-transformed amplitude, across permutations.
If we, for example, want to perform a cluster-based non-parametric analyses, we can save the largest cluster size (given some initial \(z\)-value threshold) for each iteration. Then, across our (let’s say) 5000 permutations, we have acquired a distribution of maximum cluster sizes under the null hypothesis of no effect.
We actually did this for our simulated data: we kept track of the maximum cluster size across 1000 permutations given some initial \(z\)-value cutoff. We’ll plot such a non-parametric distribution below (for an arbitrary \(z\)-value cutoff of 3):
np_dist = np.load('clust_size_dist_data.npz')
zx, clust_sizes = np_dist['zx'], np_dist['dist']
z_cutoff = 3
z_idx = np.abs(zx - z_cutoff).argmin()
clust_size_dist = clust_sizes[:, z_idx]
plt.figure(figsize=(15, 5))
plt.title("Max. cluster size across 1000 permutations", fontsize=25)
plt.hist(clust_size_dist, bins=50)
plt.xlabel("Max. cluster size", fontsize=20)
plt.ylabel("Frequency", fontsize=20)
plt.grid()
plt.show()
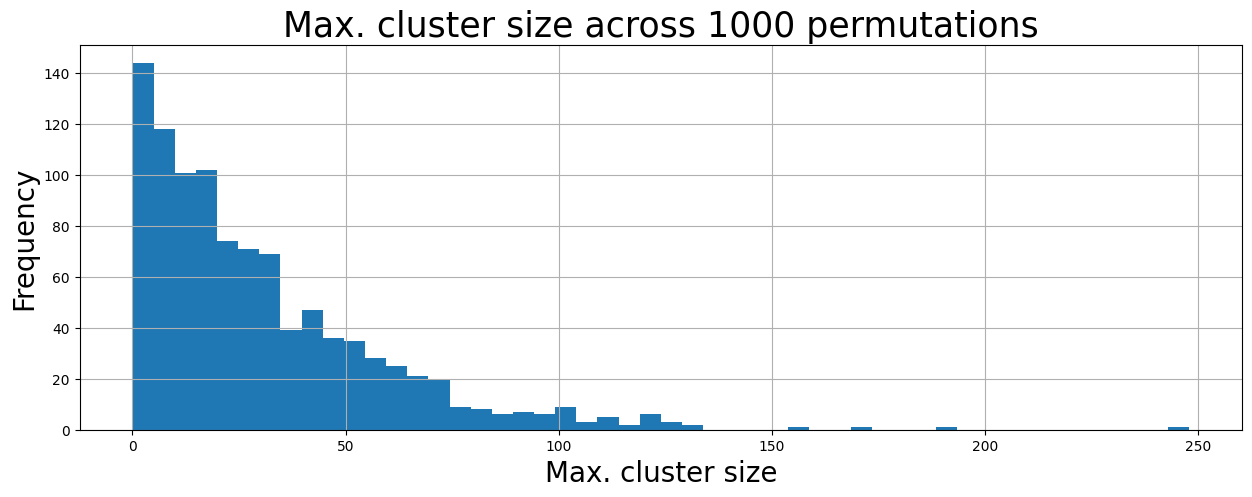
With that information, we can calculate the non-parametric \(p\)-value of each of our observed clusters using the same type of formula as we used earlier:
The same logic holds for voxel-based (TFCE-transformed) amplitude, where you wouldn’t keep track of the maximum cluster size, but the maximum amplitude across permutations.
""" Implement the (optional) ToDo here. """
# YOUR CODE HERE
raise NotImplementedError()
''' Tests the above (optional) ToDo. '''
np.testing.assert_almost_equal(pval_clust_size, 0.001998)
print("Well done!")
In our experience, non-parametric analyses (e.g., randomise
in FSL) in combination with TFCE (also supported in FSL) is a very sensitive approach, allowing for voxel-wise inference while taking into account the “blobbiness” of effects!
Exercise on new data#
Suppose we repeat the cat-picture experiment which we described earlier. Based on the literature, we expect to find strong activation in a small group of voxels — known as the nucleus felix — which is about 29 “voxels” in volume, located in the middle of the brain (here: our 2D brain). Like our other example, we’ve measured a group-level (2D) statistics (\(z\)-values) map which represents the cat-against-baseline contrast.
We’ll load in and plot the new data below:
data2 = np.load('data_assignment.npy')
plot_sim_brain(data2, title='Simulated data assignment', vmin=-10, vmax=10)
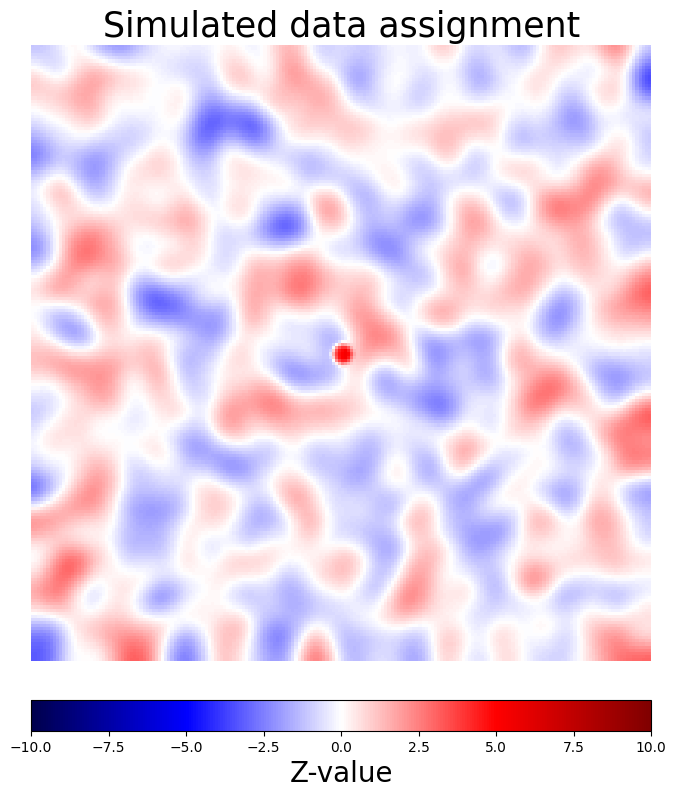
# Apply cluster-based RFT
YOUR ANSWER HERE
Effect of different MCC strategies on real data#
We actually ran group-level analyses (using FLAME1-type mixed-effects) with different MCC methods on our run-level \(4\cdot \beta_{face} - \beta_{place} - \beta_{body} - \beta_{character} - \beta_{object}\) contrast from 12 subjects, which we download below:
import os
data_dir = os.path.join(os.path.expanduser("~"), 'NI-edu-data')
print("Downloading group-level FSL FEAT results (+- 133MB) ...")
!aws s3 sync --no-sign-request s3://openneuro.org/ds003965 {data_dir} --exclude "*" --include "derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1*/*"
print("\nDone!")
Downloading group-level FSL FEAT results (+- 133MB) ...
Completed 256.0 KiB/~61.4 MiB (335.9 KiB/s) with ~273 file(s) remaining (calculating...)
Completed 512.0 KiB/~61.4 MiB (665.5 KiB/s) with ~273 file(s) remaining (calculating...)
Completed 768.0 KiB/~61.4 MiB (989.6 KiB/s) with ~273 file(s) remaining (calculating...)
Completed 1.0 MiB/~61.4 MiB (1.3 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 1.2 MiB/~61.4 MiB (1.6 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 1.5 MiB/~61.4 MiB (1.9 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 1.8 MiB/~61.4 MiB (2.2 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 2.0 MiB/~61.4 MiB (2.5 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 2.2 MiB/~61.4 MiB (2.8 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 2.5 MiB/~61.4 MiB (2.8 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 2.8 MiB/~61.4 MiB (3.1 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 3.0 MiB/~61.4 MiB (3.4 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 3.2 MiB/~61.4 MiB (3.7 MiB/s) with ~273 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.7 MiB/s) with ~273 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/bg_image.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/bg_image.nii.gz
Completed 3.3 MiB/~61.4 MiB (3.7 MiB/s) with ~272 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.6 MiB/s) with ~272 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/cluster_mask_zstat1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/cluster_mask_zstat1.nii.gz
Completed 3.3 MiB/~61.4 MiB (3.6 MiB/s) with ~271 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.6 MiB/s) with ~271 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.con to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.con
Completed 3.3 MiB/~61.4 MiB (3.6 MiB/s) with ~270 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.5 MiB/s) with ~270 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.4 MiB/s) with ~270 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.lcon to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.lcon
Completed 3.3 MiB/~61.4 MiB (3.4 MiB/s) with ~269 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat1 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat1
Completed 3.3 MiB/~61.4 MiB (3.4 MiB/s) with ~268 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.4 MiB/s) with ~268 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/cluster_zstat1_std.txt to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/cluster_zstat1_std.txt
Completed 3.3 MiB/~61.4 MiB (3.4 MiB/s) with ~267 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.4 MiB/s) with ~267 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.3 MiB/s) with ~267 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.3 MiB/s) with ~267 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.3 MiB/s) with ~267 file(s) remaining (calculating...)
Completed 3.3 MiB/~61.4 MiB (3.2 MiB/s) with ~267 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3a_flame to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3a_flame
Completed 3.3 MiB/~61.4 MiB (3.2 MiB/s) with ~266 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.mat to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.mat
Completed 3.3 MiB/~61.4 MiB (3.2 MiB/s) with ~265 file(s) remaining (calculating...)
Completed 3.4 MiB/~61.4 MiB (3.3 MiB/s) with ~265 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.lev to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.lev
Completed 3.4 MiB/~61.4 MiB (3.3 MiB/s) with ~264 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3a_flame.o18501 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3a_flame.o18501
Completed 3.4 MiB/~61.4 MiB (3.3 MiB/s) with ~263 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.grp to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.grp
Completed 3.4 MiB/~61.4 MiB (3.3 MiB/s) with ~262 file(s) remaining (calculating...)
Completed 3.4 MiB/~61.4 MiB (3.2 MiB/s) with ~262 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.ppm to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.ppm
Completed 3.4 MiB/~61.4 MiB (3.2 MiB/s) with ~261 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.fsf to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.fsf
Completed 3.4 MiB/~61.4 MiB (3.2 MiB/s) with ~260 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.10 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.10
Completed 3.4 MiB/~61.4 MiB (3.2 MiB/s) with ~259 file(s) remaining (calculating...)
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~259 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.11 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.11
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~258 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.1 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.1
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~257 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.14 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.14
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~256 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/design.png
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~255 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.12 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.12
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~254 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.17 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.17
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~253 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.13 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.13
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~252 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/cluster_zstat1_std.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/cluster_zstat1_std.html
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~251 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.2 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.2
Completed 3.5 MiB/~61.4 MiB (3.1 MiB/s) with ~250 file(s) remaining (calculating...)
Completed 3.7 MiB/~61.4 MiB (3.2 MiB/s) with ~250 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.21 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.21
Completed 3.7 MiB/~61.4 MiB (3.2 MiB/s) with ~249 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.20 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.20
Completed 3.7 MiB/~61.4 MiB (3.2 MiB/s) with ~248 file(s) remaining (calculating...)
Completed 4.0 MiB/~61.4 MiB (3.4 MiB/s) with ~248 file(s) remaining (calculating...)
Completed 4.2 MiB/~61.4 MiB (3.6 MiB/s) with ~248 file(s) remaining (calculating...)
Completed 4.2 MiB/~61.4 MiB (3.6 MiB/s) with ~248 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.19 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.19
Completed 4.2 MiB/~61.4 MiB (3.6 MiB/s) with ~247 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.16 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.16
Completed 4.2 MiB/~61.4 MiB (3.6 MiB/s) with ~246 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat0 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat0
Completed 4.2 MiB/~61.4 MiB (3.6 MiB/s) with ~245 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.18 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.18
Completed 4.2 MiB/~61.4 MiB (3.6 MiB/s) with ~244 file(s) remaining (calculating...)
Completed 4.5 MiB/~61.4 MiB (3.8 MiB/s) with ~244 file(s) remaining (calculating...)
Completed 4.7 MiB/~61.4 MiB (4.0 MiB/s) with ~244 file(s) remaining (calculating...)
Completed 5.0 MiB/~61.4 MiB (4.2 MiB/s) with ~244 file(s) remaining (calculating...)
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~244 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.15 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.15
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~243 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3a_flame.e18501 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3a_flame.e18501
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~242 file(s) remaining (calculating...)
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~242 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/lmax_zstat1_std.txt to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/lmax_zstat1_std.txt
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~241 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.27 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.27
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~240 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.24 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.24
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~239 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.28 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.28
Completed 5.2 MiB/~61.4 MiB (4.4 MiB/s) with ~238 file(s) remaining (calculating...)
Completed 5.5 MiB/~61.4 MiB (4.6 MiB/s) with ~238 file(s) remaining (calculating...)
Completed 5.7 MiB/~61.4 MiB (4.8 MiB/s) with ~238 file(s) remaining (calculating...)
Completed 6.0 MiB/~61.4 MiB (5.0 MiB/s) with ~238 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.23 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.23
Completed 6.0 MiB/~61.4 MiB (5.0 MiB/s) with ~237 file(s) remaining (calculating...)
Completed 6.2 MiB/~61.4 MiB (5.2 MiB/s) with ~237 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.22 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.22
Completed 6.2 MiB/~61.4 MiB (5.2 MiB/s) with ~236 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.26 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.26
Completed 6.2 MiB/~61.4 MiB (5.2 MiB/s) with ~235 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.30 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.30
Completed 6.2 MiB/~61.4 MiB (5.2 MiB/s) with ~234 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.31 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.31
Completed 6.2 MiB/~61.4 MiB (5.2 MiB/s) with ~233 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.25 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.25
Completed 6.2 MiB/~61.4 MiB (5.2 MiB/s) with ~232 file(s) remaining (calculating...)
Completed 6.5 MiB/~61.4 MiB (5.3 MiB/s) with ~232 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.3 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.3
Completed 6.5 MiB/~61.4 MiB (5.3 MiB/s) with ~231 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.37 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.37
Completed 6.5 MiB/~61.4 MiB (5.3 MiB/s) with ~230 file(s) remaining (calculating...)
Completed 6.7 MiB/~61.4 MiB (5.5 MiB/s) with ~230 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.35 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.35
Completed 6.7 MiB/~61.4 MiB (5.5 MiB/s) with ~229 file(s) remaining (calculating...)
Completed 7.0 MiB/~61.4 MiB (5.7 MiB/s) with ~229 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.39 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.39
Completed 7.0 MiB/~61.4 MiB (5.7 MiB/s) with ~228 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.36 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.36
Completed 7.0 MiB/~61.4 MiB (5.7 MiB/s) with ~227 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.34 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.34
Completed 7.0 MiB/~61.4 MiB (5.7 MiB/s) with ~226 file(s) remaining (calculating...)
Completed 7.2 MiB/~61.4 MiB (5.7 MiB/s) with ~226 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.32 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.32
Completed 7.2 MiB/~61.4 MiB (5.7 MiB/s) with ~225 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.38 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.38
Completed 7.2 MiB/~61.4 MiB (5.7 MiB/s) with ~224 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.41 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.41
Completed 7.2 MiB/~61.4 MiB (5.7 MiB/s) with ~223 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.29 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.29
Completed 7.2 MiB/~61.4 MiB (5.7 MiB/s) with ~222 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.33 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.33
Completed 7.2 MiB/~61.4 MiB (5.7 MiB/s) with ~221 file(s) remaining (calculating...)
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~221 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.46 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.46
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~220 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.43 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.43
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~219 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.42 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.42
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~218 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.44 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.44
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~217 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.45 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.45
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~216 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.47 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.47
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~215 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.50 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.50
Completed 7.5 MiB/~61.4 MiB (5.7 MiB/s) with ~214 file(s) remaining (calculating...)
Completed 7.7 MiB/~61.4 MiB (5.8 MiB/s) with ~214 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.5 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.5
Completed 7.7 MiB/~61.4 MiB (5.8 MiB/s) with ~213 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.4 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.4
Completed 7.7 MiB/~61.4 MiB (5.8 MiB/s) with ~212 file(s) remaining (calculating...)
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~212 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.54 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.54
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~211 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.48 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.48
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~210 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.49 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.49
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~209 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.55 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.55
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~208 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.56 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.56
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~207 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.52 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.52
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~206 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.57 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.57
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~205 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.59 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.59
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~204 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.60 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.60
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~203 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.63 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.63
Completed 8.0 MiB/~61.4 MiB (5.9 MiB/s) with ~202 file(s) remaining (calculating...)
Completed 8.2 MiB/~61.4 MiB (5.8 MiB/s) with ~202 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.64 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.64
Completed 8.2 MiB/~61.4 MiB (5.8 MiB/s) with ~201 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.65 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.65
Completed 8.2 MiB/~61.4 MiB (5.8 MiB/s) with ~200 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.6 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.6
Completed 8.2 MiB/~61.4 MiB (5.8 MiB/s) with ~199 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.58 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.58
Completed 8.2 MiB/~61.4 MiB (5.8 MiB/s) with ~198 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.66 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.66
Completed 8.2 MiB/~61.4 MiB (5.8 MiB/s) with ~197 file(s) remaining (calculating...)
Completed 8.5 MiB/~61.4 MiB (5.9 MiB/s) with ~197 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.67 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.67
Completed 8.5 MiB/~61.4 MiB (5.9 MiB/s) with ~196 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.61 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.61
Completed 8.5 MiB/~61.4 MiB (5.9 MiB/s) with ~195 file(s) remaining (calculating...)
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~195 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.40 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.40
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~194 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.69 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.69
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~193 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.51 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.51
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~192 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.70 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.70
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~191 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.68 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.68
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~190 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.72 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.72
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~189 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.53 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.53
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~188 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.7 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.7
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~187 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.73 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.73
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~186 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.62 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.62
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~185 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.71 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.71
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~184 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.74 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.74
Completed 8.7 MiB/~61.4 MiB (6.0 MiB/s) with ~183 file(s) remaining (calculating...)
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~183 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.75 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.75
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~182 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.76 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.76
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~181 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.77 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.77
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~180 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.80 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.80
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~179 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.78 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.78
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~178 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.8 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.8
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~177 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.79 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.79
Completed 9.0 MiB/~61.4 MiB (5.7 MiB/s) with ~176 file(s) remaining (calculating...)
Completed 9.2 MiB/~61.4 MiB (5.7 MiB/s) with ~176 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.81 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.81
Completed 9.2 MiB/~61.4 MiB (5.7 MiB/s) with ~175 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.85 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.85
Completed 9.2 MiB/~61.4 MiB (5.7 MiB/s) with ~174 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.87 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.87
Completed 9.2 MiB/~61.4 MiB (5.7 MiB/s) with ~173 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.83 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.83
Completed 9.2 MiB/~61.4 MiB (5.7 MiB/s) with ~172 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.84 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.84
Completed 9.2 MiB/~61.4 MiB (5.7 MiB/s) with ~171 file(s) remaining (calculating...)
Completed 9.5 MiB/~61.4 MiB (5.7 MiB/s) with ~171 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.82 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.82
Completed 9.5 MiB/~61.4 MiB (5.7 MiB/s) with ~170 file(s) remaining (calculating...)
Completed 9.7 MiB/~61.4 MiB (5.9 MiB/s) with ~170 file(s) remaining (calculating...)
Completed 10.0 MiB/~61.4 MiB (6.0 MiB/s) with ~170 file(s) remaining (calculating...)
Completed 10.2 MiB/~61.4 MiB (6.1 MiB/s) with ~170 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.89 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.89
Completed 10.2 MiB/~61.4 MiB (6.1 MiB/s) with ~169 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.88 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.88
Completed 10.2 MiB/~61.4 MiB (6.1 MiB/s) with ~168 file(s) remaining (calculating...)
Completed 10.5 MiB/~61.4 MiB (6.3 MiB/s) with ~168 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.86 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.86
Completed 10.5 MiB/~61.4 MiB (6.3 MiB/s) with ~167 file(s) remaining (calculating...)
Completed 10.7 MiB/~61.4 MiB (6.4 MiB/s) with ~167 file(s) remaining (calculating...)
Completed 11.0 MiB/~61.4 MiB (6.6 MiB/s) with ~167 file(s) remaining (calculating...)
Completed 11.0 MiB/~61.4 MiB (6.6 MiB/s) with ~167 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/example_func.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/example_func.nii.gz
Completed 11.0 MiB/~61.4 MiB (6.6 MiB/s) with ~166 file(s) remaining (calculating...)
Completed 11.2 MiB/~61.4 MiB (6.7 MiB/s) with ~166 file(s) remaining (calculating...)
Completed 11.5 MiB/~61.4 MiB (6.9 MiB/s) with ~166 file(s) remaining (calculating...)
Completed 11.5 MiB/~61.4 MiB (6.9 MiB/s) with ~166 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.91 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.91
Completed 11.5 MiB/~61.4 MiB (6.9 MiB/s) with ~165 file(s) remaining (calculating...)
Completed 11.7 MiB/~61.4 MiB (7.0 MiB/s) with ~165 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.90 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.90
Completed 11.7 MiB/~61.4 MiB (7.0 MiB/s) with ~164 file(s) remaining (calculating...)
Completed 12.0 MiB/~61.4 MiB (7.1 MiB/s) with ~164 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.1 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.1
Completed 12.0 MiB/~61.4 MiB (7.1 MiB/s) with ~163 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.9 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.e22347.9
Completed 12.0 MiB/~61.4 MiB (7.1 MiB/s) with ~162 file(s) remaining (calculating...)
Completed 12.0 MiB/~61.4 MiB (7.1 MiB/s) with ~162 file(s) remaining (calculating...)
Completed 12.2 MiB/~61.4 MiB (7.2 MiB/s) with ~162 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.12 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.12
Completed 12.2 MiB/~61.4 MiB (7.2 MiB/s) with ~161 file(s) remaining (calculating...)
Completed 12.4 MiB/~61.4 MiB (7.3 MiB/s) with ~161 file(s) remaining (calculating...)
Completed 12.4 MiB/~61.4 MiB (7.3 MiB/s) with ~161 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.13 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.13
Completed 12.4 MiB/~61.4 MiB (7.3 MiB/s) with ~160 file(s) remaining (calculating...)
Completed 12.7 MiB/~61.4 MiB (7.5 MiB/s) with ~160 file(s) remaining (calculating...)
Completed 12.9 MiB/~61.4 MiB (7.6 MiB/s) with ~160 file(s) remaining (calculating...)
Completed 13.2 MiB/~61.4 MiB (7.8 MiB/s) with ~160 file(s) remaining (calculating...)
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~160 file(s) remaining (calculating...)
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~160 file(s) remaining (calculating...)
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~160 file(s) remaining (calculating...)
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~160 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.10 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.10
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~159 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.15 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.15
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~158 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.17 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.17
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~157 file(s) remaining (calculating...)
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~157 file(s) remaining (calculating...)
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~157 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.11 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.11
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~156 file(s) remaining (calculating...)
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~156 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.14 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.14
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~155 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.19 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.19
Completed 13.4 MiB/~61.4 MiB (7.9 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 13.7 MiB/~61.4 MiB (8.0 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 13.9 MiB/~61.4 MiB (8.1 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 14.2 MiB/~61.4 MiB (8.3 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 14.4 MiB/~61.4 MiB (8.4 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 14.7 MiB/~61.4 MiB (8.6 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 14.9 MiB/~61.4 MiB (8.7 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 15.2 MiB/~61.4 MiB (8.8 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 15.4 MiB/~61.4 MiB (9.0 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 15.4 MiB/~61.4 MiB (9.0 MiB/s) with ~154 file(s) remaining (calculating...)
Completed 15.4 MiB/~61.4 MiB (9.0 MiB/s) with ~154 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.21 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.21
Completed 15.4 MiB/~61.4 MiB (9.0 MiB/s) with ~153 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.18 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.18
Completed 15.4 MiB/~61.4 MiB (9.0 MiB/s) with ~152 file(s) remaining (calculating...)
Completed 15.4 MiB/~61.4 MiB (8.9 MiB/s) with ~152 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.23 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.23
Completed 15.4 MiB/~61.4 MiB (8.9 MiB/s) with ~151 file(s) remaining (calculating...)
Completed 15.4 MiB/~61.4 MiB (8.9 MiB/s) with ~151 file(s) remaining (calculating...)
Completed 15.4 MiB/~61.4 MiB (8.9 MiB/s) with ~151 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.24 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.24
Completed 15.4 MiB/~61.4 MiB (8.9 MiB/s) with ~150 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.25 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.25
Completed 15.4 MiB/~61.4 MiB (8.9 MiB/s) with ~149 file(s) remaining (calculating...)
Completed 15.4 MiB/~61.4 MiB (8.9 MiB/s) with ~149 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (9.0 MiB/s) with ~149 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.2 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.2
Completed 15.7 MiB/~61.4 MiB (9.0 MiB/s) with ~148 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (9.0 MiB/s) with ~148 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.16 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.16
Completed 15.7 MiB/~61.4 MiB (9.0 MiB/s) with ~147 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (9.0 MiB/s) with ~147 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.22 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.22
Completed 15.7 MiB/~61.4 MiB (9.0 MiB/s) with ~146 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.9 MiB/s) with ~146 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.9 MiB/s) with ~146 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.9 MiB/s) with ~146 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.9 MiB/s) with ~146 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.8 MiB/s) with ~146 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.28 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.28
Completed 15.7 MiB/~61.4 MiB (8.8 MiB/s) with ~145 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.29 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.29
Completed 15.7 MiB/~61.4 MiB (8.8 MiB/s) with ~144 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.20 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.20
Completed 15.7 MiB/~61.4 MiB (8.8 MiB/s) with ~143 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.8 MiB/s) with ~143 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.8 MiB/s) with ~143 file(s) remaining (calculating...)
Completed 15.7 MiB/~61.4 MiB (8.7 MiB/s) with ~143 file(s) remaining (calculating...)
Completed 15.9 MiB/~61.4 MiB (8.9 MiB/s) with ~143 file(s) remaining (calculating...)
Completed 15.9 MiB/~61.4 MiB (8.8 MiB/s) with ~143 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.3 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.3
Completed 15.9 MiB/~61.4 MiB (8.8 MiB/s) with ~142 file(s) remaining (calculating...)
Completed 16.2 MiB/~61.4 MiB (8.9 MiB/s) with ~142 file(s) remaining (calculating...)
Completed 16.4 MiB/~61.4 MiB (9.1 MiB/s) with ~142 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (9.2 MiB/s) with ~142 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.26 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.26
Completed 16.7 MiB/~61.4 MiB (9.2 MiB/s) with ~141 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.27 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.27
Completed 16.7 MiB/~61.4 MiB (9.2 MiB/s) with ~140 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.30 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.30
Completed 16.7 MiB/~61.4 MiB (9.2 MiB/s) with ~139 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (9.2 MiB/s) with ~139 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.32 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.32
Completed 16.7 MiB/~61.4 MiB (9.2 MiB/s) with ~138 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (9.1 MiB/s) with ~138 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.31 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.31
Completed 16.7 MiB/~61.4 MiB (9.1 MiB/s) with ~137 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.34 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.34
Completed 16.7 MiB/~61.4 MiB (9.1 MiB/s) with ~136 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.33 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.33
Completed 16.7 MiB/~61.4 MiB (9.1 MiB/s) with ~135 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (9.0 MiB/s) with ~135 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (9.0 MiB/s) with ~135 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.35 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.35
Completed 16.7 MiB/~61.4 MiB (9.0 MiB/s) with ~134 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.39 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.39
Completed 16.7 MiB/~61.4 MiB (9.0 MiB/s) with ~133 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (8.9 MiB/s) with ~133 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (8.9 MiB/s) with ~133 file(s) remaining (calculating...)
Completed 16.7 MiB/~61.4 MiB (8.8 MiB/s) with ~133 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (9.0 MiB/s) with ~133 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.40 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.40
Completed 16.9 MiB/~61.4 MiB (9.0 MiB/s) with ~132 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.37 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.37
Completed 16.9 MiB/~61.4 MiB (9.0 MiB/s) with ~131 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.38 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.38
Completed 16.9 MiB/~61.4 MiB (9.0 MiB/s) with ~130 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.9 MiB/s) with ~130 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.9 MiB/s) with ~130 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.9 MiB/s) with ~130 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.9 MiB/s) with ~130 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.9 MiB/s) with ~130 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~130 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.4 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.4
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~129 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~129 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.45 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.45
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~128 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.42 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.42
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~127 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.36 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.36
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~126 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~126 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.43 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.43
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~125 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.47 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.47
Completed 16.9 MiB/~61.4 MiB (8.8 MiB/s) with ~124 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.7 MiB/s) with ~124 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.41 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.41
Completed 16.9 MiB/~61.4 MiB (8.7 MiB/s) with ~123 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.49 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.49
Completed 16.9 MiB/~61.4 MiB (8.7 MiB/s) with ~122 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.6 MiB/s) with ~122 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.46 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.46
Completed 16.9 MiB/~61.4 MiB (8.6 MiB/s) with ~121 file(s) remaining (calculating...)
Completed 16.9 MiB/~61.4 MiB (8.6 MiB/s) with ~121 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.44 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.44
Completed 16.9 MiB/~61.4 MiB (8.6 MiB/s) with ~120 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~120 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~120 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~120 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/filtered_func_data.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/filtered_func_data.nii.gz
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~119 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.52 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.52
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~118 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~118 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.50 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.50
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~117 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.54 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.54
Completed 17.2 MiB/~61.4 MiB (8.7 MiB/s) with ~116 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.6 MiB/s) with ~116 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.51 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.51
Completed 17.2 MiB/~61.4 MiB (8.6 MiB/s) with ~115 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.6 MiB/s) with ~115 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.53 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.53
Completed 17.2 MiB/~61.4 MiB (8.6 MiB/s) with ~114 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.6 MiB/s) with ~114 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.6 MiB/s) with ~114 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.55 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.55
Completed 17.2 MiB/~61.4 MiB (8.6 MiB/s) with ~113 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.5 MiB/s) with ~113 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.5 MiB/s) with ~113 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.5 MiB/s) with ~113 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~113 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~113 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.57 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.57
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~112 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.6 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.6
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~111 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.58 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.58
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~110 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~110 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.56 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.56
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~109 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.48 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.48
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~108 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.5 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.5
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~107 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.59 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.59
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~106 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.61 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.61
Completed 17.2 MiB/~61.4 MiB (8.4 MiB/s) with ~105 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.3 MiB/s) with ~105 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.62 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.62
Completed 17.2 MiB/~61.4 MiB (8.3 MiB/s) with ~104 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.3 MiB/s) with ~104 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.3 MiB/s) with ~104 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~104 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~104 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~104 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.60 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.60
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~103 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~103 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.69 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.69
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~102 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.64 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.64
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~101 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.65 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.65
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~100 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~100 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.66 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.66
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~99 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.70 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.70
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~98 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.7 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.7
Completed 17.2 MiB/~61.4 MiB (8.2 MiB/s) with ~97 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~97 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.63 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.63
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~96 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~96 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.71 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.71
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~95 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~95 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~95 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~95 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~95 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.73 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.73
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~94 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.72 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.72
Completed 17.2 MiB/~61.4 MiB (8.1 MiB/s) with ~93 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (8.0 MiB/s) with ~93 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~93 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.68 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.68
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~92 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.75 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.75
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~91 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~91 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~91 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~91 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~91 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~91 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.74 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.74
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~90 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~90 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~90 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.82 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.82
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~89 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.67 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.67
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~88 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.8 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.8
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~87 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.79 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.79
Completed 17.2 MiB/~61.4 MiB (7.9 MiB/s) with ~86 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~86 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.78 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.78
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~85 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.76 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.76
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~84 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.80 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.80
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~83 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.77 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.77
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~82 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~82 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.81 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.81
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~81 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~81 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.83 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.83
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~80 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.86 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.86
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~79 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~79 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.90 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.90
Completed 17.2 MiB/~61.4 MiB (7.8 MiB/s) with ~78 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~78 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.91 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.91
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~77 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~77 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~77 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.89 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.89
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~76 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3c_flame.e27595 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3c_flame.e27595
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~75 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3c_flame to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3c_flame
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~74 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~74 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.88 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.88
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~73 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~73 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3c_flame.o27595 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3c_flame.o27595
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~72 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat4_post.e28365 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat4_post.e28365
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~71 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~71 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.87 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.87
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~70 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat4_post to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat4_post
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~69 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat4_post.o28365 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat4_post.o28365
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~68 file(s) remaining (calculating...)
Completed 17.2 MiB/~61.4 MiB (7.7 MiB/s) with ~68 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~68 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat5_stop.e29217 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat5_stop.e29217
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~67 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.85 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.85
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~66 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~66 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~66 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~66 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.9 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.9
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~65 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat5_stop.o29217 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat5_stop.o29217
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~64 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/mask.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/mask.nii.gz
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~63 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.84 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat3b_flame.o22347.84
Completed 17.3 MiB/~61.4 MiB (7.6 MiB/s) with ~62 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.5 MiB/s) with ~62 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.5 MiB/s) with ~62 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.5 MiB/s) with ~62 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat9 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/logs/feat9
Completed 17.3 MiB/~61.4 MiB (7.5 MiB/s) with ~61 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.4 MiB/s) with ~61 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report_poststats.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report_poststats.html
Completed 17.3 MiB/~61.4 MiB (7.4 MiB/s) with ~60 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report_stats.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report_stats.html
Completed 17.3 MiB/~61.4 MiB (7.4 MiB/s) with ~59 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/dof to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/dof
Completed 17.3 MiB/~61.4 MiB (7.4 MiB/s) with ~58 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.4 MiB/s) with ~58 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/global_prob_outlier1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/global_prob_outlier1.nii.gz
Completed 17.3 MiB/~61.4 MiB (7.4 MiB/s) with ~57 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report.html
Completed 17.3 MiB/~61.4 MiB (7.4 MiB/s) with ~56 file(s) remaining (calculating...)
Completed 17.3 MiB/~61.4 MiB (7.3 MiB/s) with ~56 file(s) remaining (calculating...)
Completed 17.5 MiB/~61.4 MiB (7.4 MiB/s) with ~56 file(s) remaining (calculating...)
Completed 17.6 MiB/~61.4 MiB (7.4 MiB/s) with ~56 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/logfile to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/logfile
Completed 17.6 MiB/~61.4 MiB (7.4 MiB/s) with ~55 file(s) remaining (calculating...)
Completed 17.8 MiB/~61.4 MiB (7.5 MiB/s) with ~55 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report_log.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/report_log.html
Completed 17.8 MiB/~61.4 MiB (7.5 MiB/s) with ~54 file(s) remaining (calculating...)
Completed 17.8 MiB/~61.4 MiB (7.4 MiB/s) with ~54 file(s) remaining (calculating...)
Completed 17.8 MiB/~61.4 MiB (7.4 MiB/s) with ~54 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/rendered_thresh_zstat1.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/rendered_thresh_zstat1.png
Completed 17.8 MiB/~61.4 MiB (7.4 MiB/s) with ~53 file(s) remaining (calculating...)
Completed 17.8 MiB/~61.4 MiB (7.4 MiB/s) with ~53 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/mean_outlier_random_effects_var1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/mean_outlier_random_effects_var1.nii.gz
Completed 17.8 MiB/~61.4 MiB (7.4 MiB/s) with ~52 file(s) remaining (calculating...)
Completed 18.1 MiB/~61.4 MiB (7.5 MiB/s) with ~52 file(s) remaining (calculating...)
Completed 18.3 MiB/~61.4 MiB (7.6 MiB/s) with ~52 file(s) remaining (calculating...)
Completed 18.4 MiB/~61.4 MiB (7.6 MiB/s) with ~52 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/smoothness to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/smoothness
Completed 18.4 MiB/~61.4 MiB (7.6 MiB/s) with ~51 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/tdof_t1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/tdof_t1.nii.gz
Completed 18.4 MiB/~61.4 MiB (7.6 MiB/s) with ~50 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/prob_outlier1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/prob_outlier1.nii.gz
Completed 18.4 MiB/~61.4 MiB (7.6 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 18.6 MiB/~61.4 MiB (7.6 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 18.9 MiB/~61.4 MiB (7.7 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 19.1 MiB/~61.4 MiB (7.8 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 19.4 MiB/~61.4 MiB (7.8 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 19.6 MiB/~61.4 MiB (7.9 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 19.7 MiB/~61.4 MiB (7.9 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 20.0 MiB/~61.4 MiB (7.9 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 20.2 MiB/~61.4 MiB (8.0 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 20.5 MiB/~61.4 MiB (8.1 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 20.7 MiB/~61.4 MiB (8.2 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 21.0 MiB/~61.4 MiB (8.3 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 21.2 MiB/~61.4 MiB (8.4 MiB/s) with ~49 file(s) remaining (calculating...)
Completed 21.5 MiB/~61.4 MiB (8.5 MiB/s) with ~49 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/cope1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/cope1.nii.gz
Completed 21.5 MiB/~61.4 MiB (8.5 MiB/s) with ~48 file(s) remaining (calculating...)
Completed 21.6 MiB/~61.4 MiB (8.5 MiB/s) with ~48 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/pe1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/pe1.nii.gz
Completed 21.6 MiB/~61.4 MiB (8.5 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 21.8 MiB/~61.4 MiB (8.5 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 22.1 MiB/~61.4 MiB (8.6 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 22.3 MiB/~61.4 MiB (8.6 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 22.6 MiB/~61.4 MiB (8.7 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 22.8 MiB/~61.4 MiB (8.8 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 23.1 MiB/~61.4 MiB (8.9 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 23.3 MiB/~61.4 MiB (8.9 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 23.6 MiB/~61.4 MiB (9.0 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 23.8 MiB/~61.4 MiB (9.1 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 24.1 MiB/~61.4 MiB (9.2 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 24.3 MiB/~61.4 MiB (9.2 MiB/s) with ~47 file(s) remaining (calculating...)
Completed 24.4 MiB/~61.4 MiB (9.2 MiB/s) with ~47 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/varcope1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/varcope1.nii.gz
Completed 24.4 MiB/~61.4 MiB (9.2 MiB/s) with ~46 file(s) remaining (calculating...)
Completed 24.6 MiB/~61.4 MiB (9.3 MiB/s) with ~46 file(s) remaining (calculating...)
Completed 24.9 MiB/~61.4 MiB (9.4 MiB/s) with ~46 file(s) remaining (calculating...)
Completed 25.1 MiB/~61.4 MiB (9.4 MiB/s) with ~46 file(s) remaining (calculating...)
Completed 25.4 MiB/~61.4 MiB (9.5 MiB/s) with ~46 file(s) remaining (calculating...)
Completed 25.6 MiB/~61.4 MiB (9.6 MiB/s) with ~46 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/rendered_thresh_zstat1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/rendered_thresh_zstat1.nii.gz
Completed 25.6 MiB/~61.4 MiB (9.6 MiB/s) with ~45 file(s) remaining (calculating...)
Completed 25.8 MiB/~61.4 MiB (9.7 MiB/s) with ~45 file(s) remaining (calculating...)
Completed 26.1 MiB/~61.4 MiB (9.7 MiB/s) with ~45 file(s) remaining (calculating...)
Completed 26.1 MiB/~61.4 MiB (9.8 MiB/s) with ~45 file(s) remaining (calculating...)
Completed 26.2 MiB/~61.4 MiB (9.8 MiB/s) with ~45 file(s) remaining (calculating...)
Completed 26.5 MiB/~61.4 MiB (9.9 MiB/s) with ~45 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/mean_random_effects_var1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/mean_random_effects_var1.nii.gz
Completed 26.5 MiB/~61.4 MiB (9.9 MiB/s) with ~44 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/tstat1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/tstat1.nii.gz
Completed 26.5 MiB/~61.4 MiB (9.9 MiB/s) with ~43 file(s) remaining (calculating...)
Completed 26.6 MiB/~61.4 MiB (9.9 MiB/s) with ~43 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/zflame1lowertstat1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/zflame1lowertstat1.nii.gz
Completed 26.6 MiB/~61.4 MiB (9.9 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 26.8 MiB/~61.4 MiB (10.0 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 27.1 MiB/~61.4 MiB (10.1 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 27.3 MiB/~61.4 MiB (10.2 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 27.6 MiB/~61.4 MiB (10.3 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 27.8 MiB/~61.4 MiB (10.4 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 28.1 MiB/~61.4 MiB (10.5 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 28.3 MiB/~61.4 MiB (10.5 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 28.6 MiB/~61.4 MiB (10.6 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 28.8 MiB/~61.4 MiB (10.7 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 28.8 MiB/~61.4 MiB (10.7 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 28.8 MiB/~61.4 MiB (10.7 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 29.1 MiB/~61.4 MiB (10.8 MiB/s) with ~42 file(s) remaining (calculating...)
Completed 29.3 MiB/~61.4 MiB (10.9 MiB/s) with ~42 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_index to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_index
Completed 29.3 MiB/~61.4 MiB (10.9 MiB/s) with ~41 file(s) remaining (calculating...)
Completed 29.5 MiB/~61.4 MiB (10.9 MiB/s) with ~41 file(s) remaining (calculating...)
Completed 29.5 MiB/~61.4 MiB (10.9 MiB/s) with ~41 file(s) remaining (calculating...)
Completed 29.8 MiB/~61.4 MiB (11.0 MiB/s) with ~41 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tdof_filtered_func_data.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tdof_filtered_func_data.nii.gz
Completed 29.8 MiB/~61.4 MiB (11.0 MiB/s) with ~40 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/thresh_zstat1.vol to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/thresh_zstat1.vol
Completed 29.8 MiB/~61.4 MiB (11.0 MiB/s) with ~39 file(s) remaining (calculating...)
Completed 30.0 MiB/~61.4 MiB (11.1 MiB/s) with ~39 file(s) remaining (calculating...)
Completed 30.1 MiB/~61.4 MiB (11.1 MiB/s) with ~39 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_index.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_index.html
Completed 30.1 MiB/~61.4 MiB (11.1 MiB/s) with ~38 file(s) remaining (calculating...)
Completed 30.3 MiB/~61.4 MiB (11.2 MiB/s) with ~38 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/zstat1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/zstat1.nii.gz
Completed 30.3 MiB/~61.4 MiB (11.2 MiB/s) with ~37 file(s) remaining (calculating...)
Completed 30.6 MiB/~61.4 MiB (11.3 MiB/s) with ~37 file(s) remaining (calculating...)
Completed 30.8 MiB/~61.4 MiB (11.4 MiB/s) with ~37 file(s) remaining (calculating...)
Completed 30.9 MiB/~61.4 MiB (11.4 MiB/s) with ~37 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/zflame1uppertstat1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/zflame1uppertstat1.nii.gz
Completed 30.9 MiB/~61.4 MiB (11.4 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 31.2 MiB/~61.4 MiB (11.5 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 31.4 MiB/~61.4 MiB (11.6 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 31.7 MiB/~61.4 MiB (11.7 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 31.9 MiB/~61.4 MiB (11.7 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 32.2 MiB/~61.4 MiB (11.8 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 32.4 MiB/~61.4 MiB (11.9 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 32.7 MiB/~61.4 MiB (12.0 MiB/s) with ~36 file(s) remaining (calculating...)
Completed 32.8 MiB/~61.4 MiB (12.1 MiB/s) with ~36 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/thresh_zstat1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/thresh_zstat1.nii.gz
Completed 32.8 MiB/~61.4 MiB (12.1 MiB/s) with ~35 file(s) remaining (calculating...)
Completed 33.1 MiB/~61.4 MiB (12.1 MiB/s) with ~35 file(s) remaining (calculating...)
Completed 33.3 MiB/~61.4 MiB (12.2 MiB/s) with ~35 file(s) remaining (calculating...)
Completed 33.6 MiB/~61.4 MiB (12.3 MiB/s) with ~35 file(s) remaining (calculating...)
Completed 33.6 MiB/~61.4 MiB (12.3 MiB/s) with ~35 file(s) remaining (calculating...)
Completed 33.9 MiB/~61.4 MiB (12.4 MiB/s) with ~35 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/mean_func.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/mean_func.nii.gz
Completed 33.9 MiB/~61.4 MiB (12.4 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 34.1 MiB/~61.4 MiB (12.5 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 34.4 MiB/~61.4 MiB (12.6 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 34.6 MiB/~61.4 MiB (12.7 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 34.9 MiB/~61.4 MiB (12.8 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 35.1 MiB/~61.4 MiB (12.9 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 35.4 MiB/~61.4 MiB (12.9 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 35.6 MiB/~61.4 MiB (13.0 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 35.9 MiB/~61.4 MiB (13.1 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.2 MiB/s) with ~34 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.2 MiB/s) with ~34 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplotc_zstat1.txt to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplotc_zstat1.txt
Completed 36.1 MiB/~61.4 MiB (13.2 MiB/s) with ~33 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.2 MiB/s) with ~33 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.con to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.con
Completed 36.1 MiB/~61.4 MiB (13.2 MiB/s) with ~32 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.1 MiB/s) with ~32 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.1 MiB/s) with ~32 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1.png
Completed 36.1 MiB/~61.4 MiB (13.1 MiB/s) with ~31 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.0 MiB/s) with ~31 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.0 MiB/s) with ~31 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (13.0 MiB/s) with ~31 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1.html
Completed 36.1 MiB/~61.4 MiB (13.0 MiB/s) with ~30 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1p.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1p.png
Completed 36.1 MiB/~61.4 MiB (13.0 MiB/s) with ~29 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1.txt to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplot_zstat1.txt
Completed 36.1 MiB/~61.4 MiB (13.0 MiB/s) with ~28 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (12.8 MiB/s) with ~28 file(s) remaining (calculating...)
Completed 36.1 MiB/~61.4 MiB (12.8 MiB/s) with ~28 file(s) remaining (calculating...)
Completed 36.4 MiB/~61.4 MiB (12.9 MiB/s) with ~28 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplotc_zstat1p.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplotc_zstat1p.png
Completed 36.4 MiB/~61.4 MiB (12.9 MiB/s) with ~27 file(s) remaining (calculating...)
Completed 36.6 MiB/~61.4 MiB (12.9 MiB/s) with ~27 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplotc_zstat1.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/tsplot/tsplotc_zstat1.png
Completed 36.6 MiB/~61.4 MiB (12.9 MiB/s) with ~26 file(s) remaining (calculating...)
Completed 36.6 MiB/~61.4 MiB (12.9 MiB/s) with ~26 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.grp to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.grp
Completed 36.6 MiB/~61.4 MiB (12.9 MiB/s) with ~25 file(s) remaining (calculating...)
Completed 36.6 MiB/~61.4 MiB (12.8 MiB/s) with ~25 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.lcon to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.lcon
Completed 36.6 MiB/~61.4 MiB (12.8 MiB/s) with ~24 file(s) remaining (calculating...)
Completed 36.9 MiB/~61.4 MiB (12.9 MiB/s) with ~24 file(s) remaining (calculating...)
Completed 36.9 MiB/~61.4 MiB (12.9 MiB/s) with ~24 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.fsf to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.fsf
Completed 36.9 MiB/~61.4 MiB (12.9 MiB/s) with ~23 file(s) remaining (calculating...)
Completed 36.9 MiB/~61.4 MiB (12.8 MiB/s) with ~23 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.png
Completed 36.9 MiB/~61.4 MiB (12.8 MiB/s) with ~22 file(s) remaining (calculating...)
Completed 36.9 MiB/~61.4 MiB (12.7 MiB/s) with ~22 file(s) remaining (calculating...)
Completed 37.2 MiB/~61.4 MiB (12.8 MiB/s) with ~22 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design_cov.ppm to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design_cov.ppm
Completed 37.2 MiB/~61.4 MiB (12.8 MiB/s) with ~21 file(s) remaining (calculating...)
Completed 37.2 MiB/~61.4 MiB (12.7 MiB/s) with ~21 file(s) remaining (calculating...)
Completed 37.4 MiB/~61.4 MiB (12.8 MiB/s) with ~21 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.mat to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.mat
Completed 37.4 MiB/~61.4 MiB (12.8 MiB/s) with ~20 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design_cov.png to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design_cov.png
Completed 37.4 MiB/~61.4 MiB (12.8 MiB/s) with ~19 file(s) remaining (calculating...)
Completed 37.7 MiB/~61.4 MiB (12.8 MiB/s) with ~19 file(s) remaining (calculating...)
Completed 37.8 MiB/~61.4 MiB (12.8 MiB/s) with ~19 file(s) remaining (calculating...)
Completed 38.0 MiB/~61.4 MiB (12.8 MiB/s) with ~19 file(s) remaining (calculating...)
Completed 38.3 MiB/~61.4 MiB (12.9 MiB/s) with ~19 file(s) remaining (calculating...)
Completed 38.3 MiB/~61.4 MiB (12.9 MiB/s) with ~19 file(s) remaining (calculating...)
Completed 38.3 MiB/~61.4 MiB (12.9 MiB/s) with ~19 file(s) remaining (calculating...)
Completed 38.5 MiB/~61.4 MiB (13.0 MiB/s) with ~19 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.ppm to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/design.ppm
Completed 38.5 MiB/~61.4 MiB (13.0 MiB/s) with ~18 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat2_pre.e14992 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat2_pre.e14992
Completed 38.5 MiB/~61.4 MiB (13.0 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 38.5 MiB/~61.4 MiB (12.9 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 38.8 MiB/~61.4 MiB (13.0 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 39.0 MiB/~61.4 MiB (13.1 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 39.3 MiB/~61.4 MiB (13.1 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 39.5 MiB/~61.4 MiB (13.2 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 39.8 MiB/~61.4 MiB (13.3 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 40.0 MiB/~61.4 MiB (13.4 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 40.3 MiB/~61.4 MiB (13.5 MiB/s) with ~17 file(s) remaining (calculating...)
Completed 40.5 MiB/~61.4 MiB (13.5 MiB/s) with ~17 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat2_pre to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat2_pre
Completed 40.5 MiB/~61.4 MiB (13.5 MiB/s) with ~16 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat0 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat0
Completed 40.5 MiB/~61.4 MiB (13.5 MiB/s) with ~15 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat2_pre.o14992 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat2_pre.o14992
Completed 40.5 MiB/~61.4 MiB (13.5 MiB/s) with ~14 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat1 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat1
Completed 40.5 MiB/~61.4 MiB (13.5 MiB/s) with ~13 file(s) remaining (calculating...)
Completed 40.8 MiB/~61.4 MiB (13.6 MiB/s) with ~13 file(s) remaining (calculating...)
Completed 41.0 MiB/~61.4 MiB (13.6 MiB/s) with ~13 file(s) remaining (calculating...)
Completed 41.0 MiB/~61.4 MiB (13.6 MiB/s) with ~13 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat5_stop.e29392 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat5_stop.e29392
Completed 41.0 MiB/~61.4 MiB (13.6 MiB/s) with ~12 file(s) remaining (calculating...)
Completed 41.3 MiB/~61.4 MiB (13.6 MiB/s) with ~12 file(s) remaining (calculating...)
Completed 41.5 MiB/~61.4 MiB (13.7 MiB/s) with ~12 file(s) remaining (calculating...)
Completed 41.8 MiB/~61.4 MiB (13.7 MiB/s) with ~12 file(s) remaining (calculating...)
Completed 42.0 MiB/~61.4 MiB (13.8 MiB/s) with ~12 file(s) remaining (calculating...)
Completed 42.0 MiB/~61.4 MiB (13.7 MiB/s) with ~12 file(s) remaining (calculating...)
Completed 42.3 MiB/~61.4 MiB (13.8 MiB/s) with ~12 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat5_stop.o29392 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat5_stop.o29392
Completed 42.3 MiB/~61.4 MiB (13.8 MiB/s) with ~11 file(s) remaining (calculating...)
Completed 42.5 MiB/~61.4 MiB (13.9 MiB/s) with ~11 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat9 to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/logs/feat9
Completed 42.5 MiB/~61.4 MiB (13.9 MiB/s) with ~10 file(s) remaining (calculating...)
Completed 42.6 MiB/~61.4 MiB (13.9 MiB/s) with ~10 file(s) remaining (calculating...)
Completed 42.6 MiB/~61.4 MiB (13.8 MiB/s) with ~10 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_firstlevel.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_firstlevel.html
Completed 42.6 MiB/~61.4 MiB (13.8 MiB/s) with ~9 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report.html
Completed 42.6 MiB/~61.4 MiB (13.8 MiB/s) with ~8 file(s) remaining (calculating...)
Completed 42.8 MiB/~61.4 MiB (13.8 MiB/s) with ~8 file(s) remaining (calculating...)
Completed 43.1 MiB/~61.4 MiB (13.9 MiB/s) with ~8 file(s) remaining (calculating...)
Completed 43.3 MiB/~61.4 MiB (14.0 MiB/s) with ~8 file(s) remaining (calculating...)
Completed 43.6 MiB/~61.4 MiB (14.1 MiB/s) with ~8 file(s) remaining (calculating...)
Completed 43.6 MiB/~61.4 MiB (14.0 MiB/s) with ~8 file(s) remaining (calculating...)
Completed 43.6 MiB/~61.4 MiB (14.0 MiB/s) with ~8 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/mask.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/mask.nii.gz
Completed 43.6 MiB/~61.4 MiB (14.0 MiB/s) with ~7 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/weights1.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/weights1.nii.gz
Completed 43.6 MiB/~61.4 MiB (14.0 MiB/s) with ~6 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_log.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_log.html
Completed 43.6 MiB/~61.4 MiB (14.0 MiB/s) with ~5 file(s) remaining (calculating...)
Completed 43.6 MiB/~61.4 MiB (13.9 MiB/s) with ~5 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_reg.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_reg.html
Completed 43.6 MiB/~61.4 MiB (13.9 MiB/s) with ~4 file(s) remaining (calculating...)
Completed 43.8 MiB/~61.4 MiB (13.9 MiB/s) with ~4 file(s) remaining (calculating...)
Completed 44.1 MiB/~61.4 MiB (14.0 MiB/s) with ~4 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_stats.html to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/report_stats.html
Completed 44.1 MiB/~61.4 MiB (14.0 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 44.3 MiB/~61.4 MiB (14.0 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 44.6 MiB/~61.4 MiB (14.1 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 44.8 MiB/~61.4 MiB (14.2 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 45.1 MiB/~61.4 MiB (14.2 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 45.3 MiB/~61.4 MiB (14.3 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 45.6 MiB/~61.4 MiB (14.4 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 45.6 MiB/~61.4 MiB (14.4 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 45.9 MiB/~61.4 MiB (14.5 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 46.1 MiB/~61.4 MiB (14.6 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 46.4 MiB/~61.4 MiB (14.6 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 46.6 MiB/~61.4 MiB (14.7 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 46.9 MiB/~61.4 MiB (14.8 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 47.1 MiB/~61.4 MiB (14.9 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 47.4 MiB/~61.4 MiB (14.9 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 47.6 MiB/~61.4 MiB (15.0 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 47.9 MiB/~61.4 MiB (15.1 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 48.1 MiB/~61.4 MiB (15.2 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 48.4 MiB/~61.4 MiB (15.2 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 48.6 MiB/~61.4 MiB (15.3 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 48.9 MiB/~61.4 MiB (15.4 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 49.1 MiB/~61.4 MiB (15.5 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 49.4 MiB/~61.4 MiB (15.5 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 49.6 MiB/~61.4 MiB (15.6 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 49.9 MiB/~61.4 MiB (15.7 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 50.1 MiB/~61.4 MiB (15.8 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 50.4 MiB/~61.4 MiB (15.8 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 50.6 MiB/~61.4 MiB (15.9 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 50.9 MiB/~61.4 MiB (16.0 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 51.1 MiB/~61.4 MiB (16.1 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 51.4 MiB/~61.4 MiB (16.1 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 51.6 MiB/~61.4 MiB (16.2 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 51.9 MiB/~61.4 MiB (16.3 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 52.1 MiB/~61.4 MiB (16.3 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 52.4 MiB/~61.4 MiB (16.4 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 52.6 MiB/~61.4 MiB (16.5 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 52.9 MiB/~61.4 MiB (16.6 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 53.1 MiB/~61.4 MiB (16.6 MiB/s) with ~3 file(s) remaining (calculating...)
Completed 53.2 MiB/~61.4 MiB (16.6 MiB/s) with ~3 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/mean_func.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/mean_func.nii.gz
Completed 53.2 MiB/~61.4 MiB (16.6 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 53.4 MiB/~61.4 MiB (16.7 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 53.7 MiB/~61.4 MiB (16.8 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 53.9 MiB/~61.4 MiB (16.9 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 54.2 MiB/~61.4 MiB (16.9 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 54.4 MiB/~61.4 MiB (17.0 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 54.7 MiB/~61.4 MiB (17.1 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 54.9 MiB/~61.4 MiB (17.2 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 55.2 MiB/~61.4 MiB (17.2 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 55.4 MiB/~61.4 MiB (17.3 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 55.7 MiB/~61.4 MiB (17.4 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 55.9 MiB/~61.4 MiB (17.5 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 56.2 MiB/~61.4 MiB (17.5 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 56.4 MiB/~61.4 MiB (17.6 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 56.7 MiB/~61.4 MiB (17.7 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 56.9 MiB/~61.4 MiB (17.8 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 57.2 MiB/~61.4 MiB (17.8 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 57.4 MiB/~61.4 MiB (17.9 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 57.7 MiB/~61.4 MiB (18.0 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 57.9 MiB/~61.4 MiB (18.1 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 58.2 MiB/~61.4 MiB (18.1 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 58.4 MiB/~61.4 MiB (18.2 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 58.7 MiB/~61.4 MiB (18.3 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 58.9 MiB/~61.4 MiB (18.4 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 59.2 MiB/~61.4 MiB (18.4 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 59.4 MiB/~61.4 MiB (18.5 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 59.7 MiB/~61.4 MiB (18.6 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 59.9 MiB/~61.4 MiB (18.6 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 60.2 MiB/~61.4 MiB (18.7 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 60.4 MiB/~61.4 MiB (18.8 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 60.7 MiB/~61.4 MiB (18.9 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 60.9 MiB/~61.4 MiB (18.9 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 61.2 MiB/~61.4 MiB (19.0 MiB/s) with ~2 file(s) remaining (calculating...)
Completed 61.4 MiB/~61.4 MiB (19.1 MiB/s) with ~2 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/res4d.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/stats/res4d.nii.gz
Completed 61.4 MiB/~61.4 MiB (19.1 MiB/s) with ~1 file(s) remaining (calculating...)
download: s3://openneuro.org/ds003965/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/var_filtered_func_data.nii.gz to ../../../../../../NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat/cope1.feat/var_filtered_func_data.nii.gz
Completed 61.4 MiB/~61.4 MiB (19.1 MiB/s) with ~0 file(s) remaining (calculating...)
Done!
from glob import glob
fsl_deriv_dir = os.path.join(data_dir, 'derivatives', 'fsl')
gl_paths = sorted(glob(os.path.join(fsl_deriv_dir, 'grouplevel_task-flocBLOCKED', '*')))
print('\n'.join(gl_paths))
/home/runner/NI-edu-data/derivatives/fsl/grouplevel_task-flocBLOCKED/contrast-faceGTother_method-FLAME1_thresh-uncorr05.gfeat
As you can see, there are results for three different MCC strategies:
uncorrected (with \(p < 0.05\));
cluster-based (with \(z > 3.1\) and \(p_{\mathrm{cluster}} < 0.05\));
voxel-based RFT (with \(p_{\mathrm{voxel}} > 0.05\));
non-parametric (“randomise” with TFCE, non-parametric \(p < 0.05\))
Let’s take a look at the thresholded \(z\)-statistic maps for each of those analyses.
You have seen this brain map before, and you should know by now that this brain map likely contains many false positives as it’s not corrected for multiple comparisons.
Here, you see a much more modest effect, where only a couple of clusters (in the superior temporal gyrus and posterior cingulate cortex) survived.
If you don’t see any green voxels, that’s right! No voxel “survived” the relatively conservative voxel-based RFT tresholding!
This looks quite alright (in the sense that at least some voxels survive the MCC procedure)! Does this mean that we should always use cluster-based or non-parametric (TFCE-boosted) MCC? Not necessarily. Like always, this depends on your data, the effect you expect, and the conclusions that you want to draw from your results.